Introduction: Scanner Light and Arduino As an RGB Lamp
Hi people!
This time I'm going to show you how to control the light from a old/broken scanner/printer. Maybe you will see, I like to get many things from junk and use in my projects.
Sometime ago I won a old printer from a teacher. Generally when I get something junky, I disassemble them and keep the things that maybe be used for something. From that printer I salvaged the motors, their mechanisms, the source, the scanner sensor and some other little things.
When i got the scanner sensor did not thought of something nice to do, but when scanning some things to study on another printer, I saw that that the scanner had his color changing (yeah, i know,i should leave the cover lowered), and remembered of an article on internet saying this sensors have a RGB led inside them.
Nice! I just had to find where I put the sensor, could be easy....
Step 1: Discovering How to Connect the RGB LED
With the sensor in hands, i had to know how to connect the LED inside. First I had to separate the pcb from the black plastic enclosure( be careful to not break it), then testing with a multimeter I was capable to see that the LED is directly connected to the header, no resistor or nothing, just each pin connected to an LED's terminal. I also discovered that the RGB LED is common anode, this means that its positive terminal is shared to each color and to make a color glow we have to connect the respective cathode(negative terminal) to ground.
Step 2: Connecting the Scanner Light and Arduino.
Since the RGB LED inside the sensor still is a LED, it was connected in the usual way to Arduino, the relative pins at each color must be connected to PWM pins, so we can select the amount of red, green and blue we want the lamp show, the only difference is that this LED is common anode and must have its common pin connected to the 5V instead of GND, and to light one of the colors the relative pin to that color should be put in LOW.
In the first attempt i used 330 ohm resistors in each cathode pin, but this, for an unknown reason didn't work, so I connected the Arduino Nano PWM pins 9, 10 and 11 directly to each LED cathode terminal, and the anode to 5V, this worked fine.
Step 3: The Code.
So, with the scanner light and the Arduino connected we only need a little piece of code to make it glow with nice colors. The code is below, it's well commented, but in next step I will speak a little about how it makes the colors shine.
//Written by Robson Couto //December 2014 //www.dragaosemchama.com.br //pins definitions #define blue 9 #define red 10 #define green 11 int bluevalue,greenvalue,redvalue,i,j,k; //variables to keep the values // of the waves in each pin void setup(){ pinMode(blue,OUTPUT);//LED pins as output pinMode(red,OUTPUT); pinMode(green,OUTPUT); redvalue=128; //first color -> red+green=yellow greenvalue=128; bluevalue=0; i=-1; j=1; k=0; } void loop(){ redvalue=redvalue+i;//changing the wave in each pin every loop cycle greenvalue=greenvalue+j; bluevalue=bluevalue+k; analogWrite(red,255-redvalue);//updating the PWM values analogWrite(green,255-greenvalue); analogWrite(blue,255-bluevalue); delay(50);//this can be changed to make the colors change faster or slower if(redvalue==255){ //test if an clor will be increased, decreased or turned off. i=-1; //see the image in the instructable to understand better. j=1; k=0; } if(greenvalue==255){ i=0; j=-1; k=1; } if(bluevalue==255){ i=1; j=0; k=-1; } }
Step 4: Understanding How This Code Works.
What the code does is change between the colors red, green and blue, mixing two colors most of the time, for example see the first image above, when the colors red and blue have the same amount the resulting color is magenta, then blue is decreased and red incread until its maximum resulting only the color red, after that red starts to decrease and green starts to increase while blue is turned off resulting in yellow.
See the second image above to know the resulting color when red, green and blue are mixed.
To change the amount of red, green and blue in the LED, the analogWrite() function is used. To use analogWrite() you need a PWM pin, so each pin of the LED, minus the anode(that is connected to +5V), needs to be conected in a PWM pin. On my Nano I used pins 9,10 e 11.
Another thing, as the LED is common anode, using analogWrite(255) on a pin will turn off that color instead of making it glow, this is because that there is no potential difference between the LED terminals, so to make a color glow in its max is necessary to use analogWrite(0).
Step 5: Make It Glow!
Well done!
Now your have an all-new RGB lamp!
Thanks for reading, see you on another instructable.
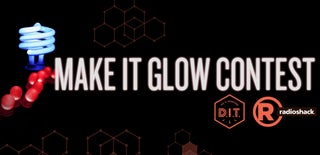
Participated in the
Make it Glow!